🚀 Introduction
Strings are crucial components of any programming language, including C++. Understanding what strings are and how to use them efficiently is critical if you wish to store text, manipulate it, or accept keyboard inputs and outputs.
This tutorial will teach you everything you need to know about string handling and working in C++.
❓ What is String in C++?
C++ defines a method for representing a sequence of characters as a class object. This class is known as `std::string`. The string class holds characters as a sequence of bytes, with the ability to access single-byte characters.
Strings are most typically employed in programs that require us to operate with text. In C++, we may execute a variety of operations on strings. For example, reversing, concatenating, or passing a function argument.
🎯 Two Kinds of Strings in C++:
- C-style Strings (C-string)
- The Standard C++ Library string class (`std::string`)
🔧 C-Style Strings
The C-style String was invented in the C language and is still supported in C++. This string is a one-dimensional array of characters that ends with the null character `\0`. Thus, a null-terminated string comprises the string's characters followed by a null.
The size of the character array holding the string must be one greater than the total number of characters in the word to accommodate the null character at the very end.
Syntax for C-style String
char name[6] = {'L', 'o', 'g', 'i', 'c', '\0'};
Alternatively, you can use a string literal, and C++ will automatically add the null character:
char name[] = "Logic"; // Size is 6 (5 letters + null terminator)
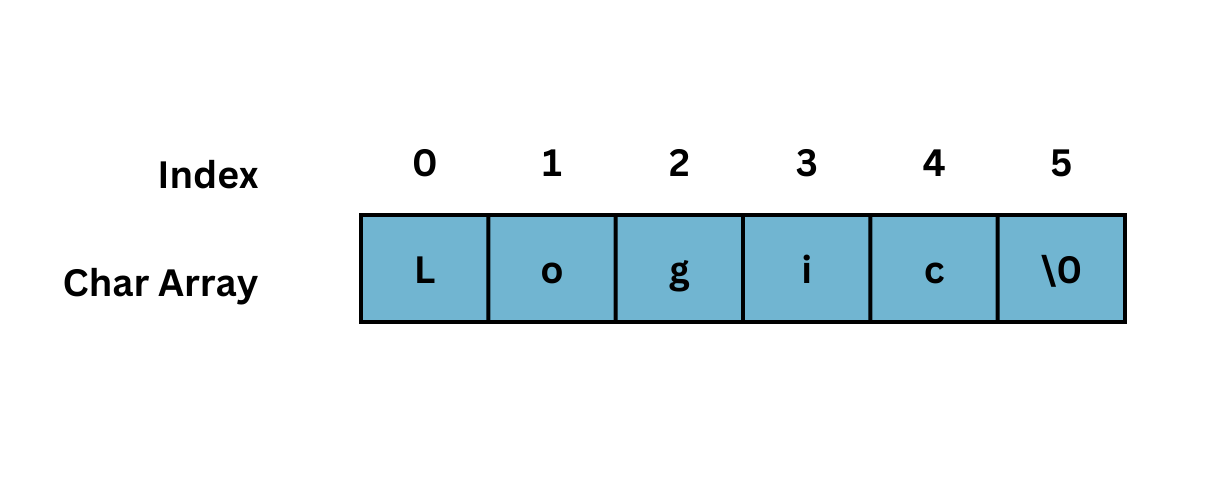
Example Program
#include <iostream>
int main() {
char name[] = "Logic";
std::cout << "Name message: " << name << std::endl;
return 0;
}
🎯 Output
Name message: Logic
⚙️ C-Style String Functions
The C Standard Library, included via the `<cstring>` header, offers functions for manipulating C-style strings.
Function | Explanation |
---|---|
`strcpy(s1, s2)` | Copies string s2 into string s1. |
`strcat(s1, s2)` | Concatenates string s2 onto the end of string s1. |
`strlen(s1)` | Returns the length of string s1. |
`strcmp(s1, s2)` | Returns 0 if s1 and s2 are the same; < 0 if s1 < s2; > 0 if s1 > s2. |
`strchr(s1, ch)` | Returns a pointer to the first occurrence of character ch in string s1. |
`strstr(s1, s2)` | Returns a pointer to the first occurrence of string s2 in string s1. |
⚡ String vs. Character Array
`std::string` | Character Array (C-style) |
---|---|
An object that represents a sequence of characters. | A collection of characters finished with a null character. |
Memory is allocated dynamically and managed automatically. | Memory must be allocated statically and managed manually. |
Slower due to overhead of dynamic memory and object management. | Faster implementation with direct memory access. |
Provides many built-in functions for powerful string operations. | Limited set of functions available from the `<cstring>` library. |
No risk of array decay when passed to functions. | Can decay to a pointer, losing size information. |
🎯 The `std::string` Class
C-style strings can be risky. If not properly null-terminated, they can cause bugs and security vulnerabilities. The `std::string` class from the C++ Standard Library is a much safer and more powerful alternative.
#include <iostream>
#include <string> // Include the C++ Standard String Class
int main() {
std::string name = "LogicMojo";
std::cout << name << "\n"; // Prints "LogicMojo"
return 0;
}
🔗 String Concatenation
String concatenation is the process of joining two or more strings. With `std::string`, this is easily done with the `+` operator.
#include <iostream>
#include <string>
int main() {
std::string str1 = "Logic";
std::string str2 = "Mojo";
std::string result = str1 + str2; // Concatenation
std::cout << result << std::endl;
return 0;
}
🎯 Output
LogicMojo
🔍 Comparing Strings
You can easily compare `std::string` objects using standard comparison operators like `==`, `!=`, `<`, `>`.
#include <iostream>
#include <string>
int main () {
std::string str1 = "hello";
std::string str2 = "world";
if (str1 == str2) {
std::cout << "Strings are equal!" << std::endl;
} else {
std::cout << "Strings are not equal." << std::endl;
}
return 0;
}
🎯 Output
Strings are not equal.
📏 Getting String Length
To get the number of characters in a `std::string`, you can use the `.length()` or `.size()` member functions (they are equivalent).
#include <iostream>
#include <string>
int main() {
std::string my_string = "This is a C++ String article";
std::cout << "Length of String = " << my_string.length() << std::endl;
return 0;
}
🎯 Output
Length of String = 28
🛠️ Common `std::string` Functions
The C++ string class includes a plethora of useful built-in functions.
Function | Description |
---|---|
`append()` | Appends to the string. |
`assign()` | Assigns a new value to the string. |
`at()` | Accesses the character at a specific position with bounds checking. |
`begin()` / `end()` | Returns iterators to the beginning/end of the string. |
`c_str()` | Returns a pointer to a C-style string representation. |
`clear()` | Erases all characters from the string. |
`compare()` | Compares the string with another string. |
`empty()` | Checks if the string is empty. |
`erase()` | Removes characters from the string. |
`find()` | Finds the first occurrence of a substring. |
`insert()` | Inserts characters at a specific position. |
`length()` / `size()` | Returns the number of characters in the string. |
`push_back()` | Appends a character to the end of the string. |
`replace()` | Replaces a portion of the string. |
`substr()` | Returns a new string that is a substring of the object. |
📤 Passing Strings to Functions
You can pass `std::string` objects to functions just like any other variable. It's often best to pass them by `const` reference (`const std::string&`) to avoid making unnecessary copies.
#include <iostream>
#include <string>
// Pass by const reference to avoid copying
void display(const std::string& s) {
std::cout << "The string is: " << s << std::endl;
}
int main() {
std::string str1;
std::cout << "Enter a string: ";
std::getline(std::cin, str1); // Read a full line
display(str1);
return 0;
}
💡 When to Prefer C-Style Strings
While `std::string` is generally superior, there are niche scenarios where C-style strings might be considered:
- Low-Level Programming: When interfacing with C libraries or hardware that expect C-style strings.
- Performance-Critical Code: In extreme cases where the overhead of `std::string`'s dynamic memory allocation is unacceptable. This is rare in modern systems.
- Legacy Codebases: When working with older C++ code that already uses C-style strings extensively.
🎉 Conclusion
This article taught you everything there is to know about the C++ String class, character arrays, and the differences between the two. You've also gone over some of the most important in-built String class functions, as well as examples, that allow you to do various actions on strings.
In C++, you can now use several functions to work with texts and strings. Learning to work with C++ strings is vital because your software may require you to take numerous inputs and produce results.
Good luck and happy learning!
❓ Frequently Asked Questions
In C++, a string is an object of the `std::string` class that represents a sequence of characters. It provides a safe and convenient way to work with text, offering many functions for operations like concatenation, searching, and modification.
The two main types are C-style strings (null-terminated character arrays) and the C++ `std::string` class. `std::string` is the modern, recommended approach due to its automatic memory management and rich feature set.
You must first include the `
std::string myString = "Hello, World!";
The key differences are:
- Representation: `std::string` is a class object; a C-string is a character array.
- Memory Management: `std::string` manages its own memory dynamically. C-strings require manual memory management.
- Functionality: `std::string` has a rich set of member functions, while C-strings rely on functions from the `
` library. - Safety: `std::string` is much safer, preventing common errors like buffer overflows.