1. What is C++?
C++ is an object-oriented programming language created by Bjarne Stroustrup.
It was released in 1985. C++ is a superset of C with the major addition of classes in C language. Initially, Stroustrup called the new language "C with classes". However,
after sometime the name was changed to C++. The idea of C++ comes from the C increment operator ++
2. What are the advantages of C++?
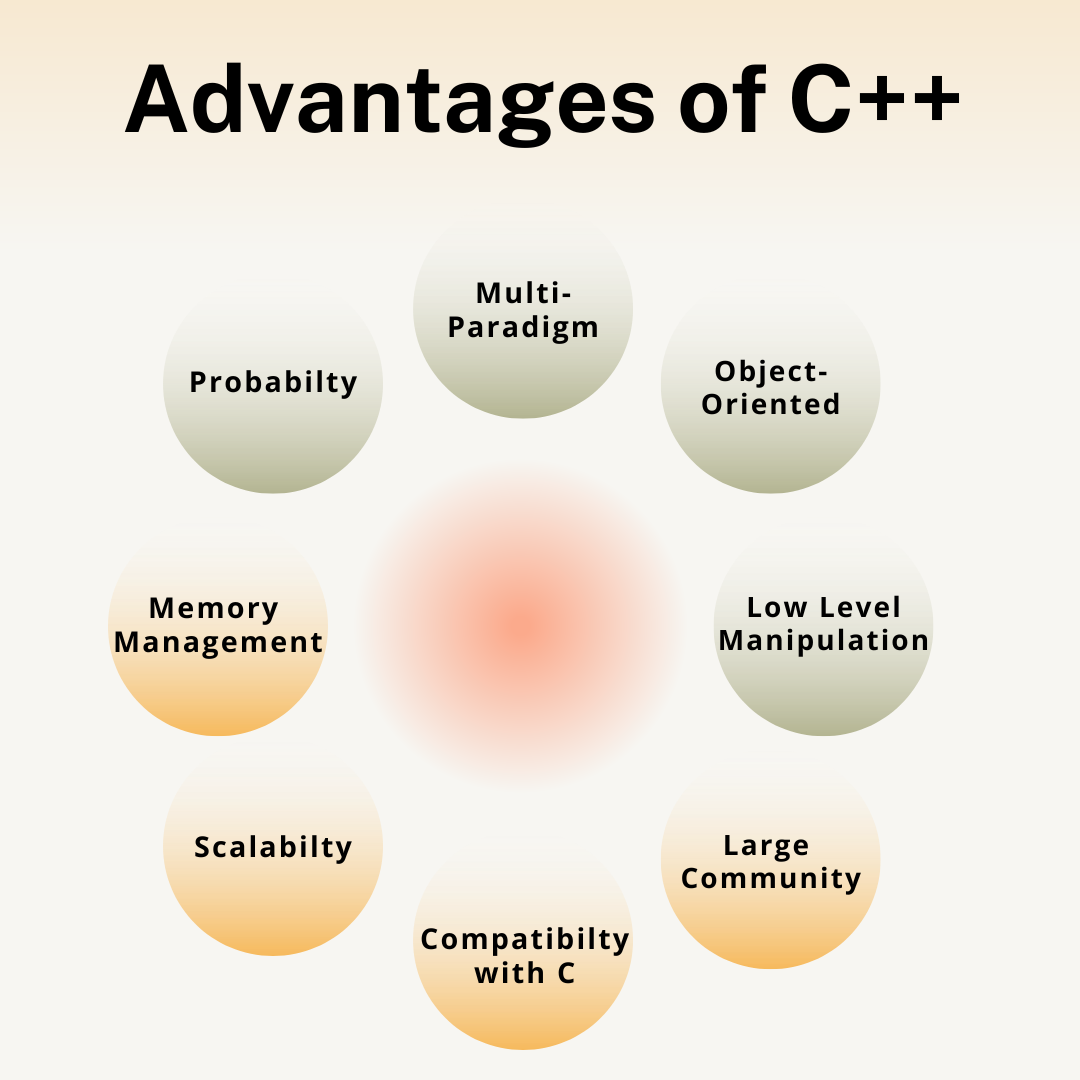
C++ doesn't only maintains all aspects from C language, it also simplifies memory management and adds several features like:
• C++ is a highly portable language means that the software developed using C++ language can run on any platform.
• C++ is an object-oriented programming language which includes the concepts such as classes, objects, inheritance, polymorphism, abstraction.
• C++ has the concept of inheritance. Through inheritance, one can eliminate the redundant code and can reuse the existing classes.
• Data hiding helps the programmer to build secure programs so that the program cannot be attacked by the invaders.
• C++ contains a rich function library.
3. What is the difference between C and C++?
Following are the differences between C and C++:
C | C++ |
---|---|
C language was developed by Dennis Ritchie. | C++ language was developed by Bjarne Stroustrup. |
C is a structured programming language. | C++ supports both structural and object-oriented programming language. |
C is a subset of C++. | C++ is a superset of C. |
C does not support the data hiding. Therefore, the data can be used by the outside world. | C++ supports data hiding. Therefore, the data cannot be accessed by the outside world. |
C supports neither function nor operator overloading. | C++ supports both function and operator overloading. |
Reference variables are not supported in C language. | C++ supports the reference variables. |
4. What is the difference between reference and pointer?
Reference | Pointer |
---|---|
Reference behaves like an alias for an existing variable, i.e., it is a temporary variable. | The pointer is a variable which stores the address of a variable. |
Reference variable does not require any indirection operator to access the value. A reference variable can be used directly to access the value. | Pointer variable requires an indirection operator to access the value of a variable. |
Once the reference variable is assigned, then it cannot be reassigned with different address values. | The pointer variable is an independent variable means that it can be reassigned to point to different objects. |
A null value cannot be assigned to the reference variable. | A null value can be assigned to the reference variable. |
5. What are the different data types present in C++?
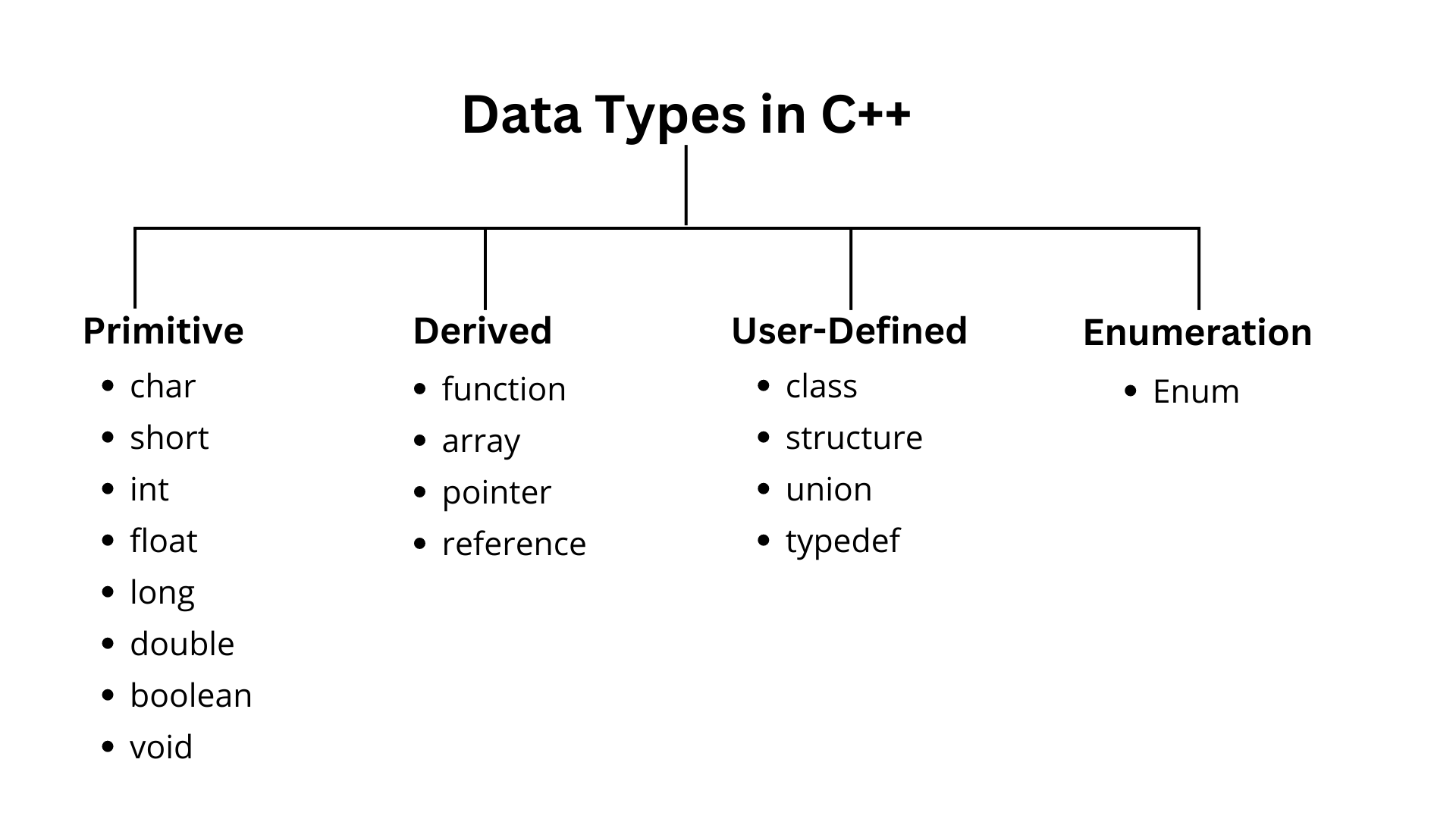
The 4 data types in C++ are given below:
• Primitive Datatype(basic datatype). Example- char, short, int, float, long, double, bool, etc.
• Derived datatype. Example- array, pointer, etc.
• Enumeration. Example- enum
• User-defined data types. Example- structure, class, etc
6. What is namespace in C++?
If there are two or more functions with the same name defined in different libraries then how will the compiler know which one to refer to? Thus namespace came to picture. A namespace defines a scope and differentiates functions, classes, variables etc. with the same name available in different libraries. The namespace starts with the keyword “namespace”. The syntax for the same is as follows:
namespace namespace_name {
// code declarations
}
7. What is template in C++?
A template in C++ is used to pass data types as parameters . These make it easier and more simpler to use classes and functions.
template(class type)ret-type func-name(parameter list){
//body of the function
}
8. What is pointer in C++?
Pointers in C++ are a data type that store the memory address of another variable.
Pointer Declaration Syntax
datatype *variable_name;
9. What is function in C++?
A function in C++ is a group of statements that together perform a specific task. Every C/C++ program has at least one function that the name is main.
The main function is called by the operating system by which our code is executed.
Syntax:
Return_type Function_name (Parameters){
// Function Body
}
10. What is destructor in C++?
Destructors in c++ are special function/methods that are used to remove memory allocation for objects. They are called usually when the scope of an object ends. eg. when a function ends you can call a destructor. They are of the same name as the class – syntax – ~(classname)();
11. Explain what is the use of void main () in C++ language?
To run the C++ application it involves two steps, the first step is a compilation where conversion of C++ code to object code take place. While second step includes linking, where combining of object code from the programmer and from libraries takes place. This function is operated by main () in C++ language.
12. Define inline function?
If a function is inline, the compiler places a copy of the code of that function at each point where the function is called at compile time. One of the important advantages of using an inline function is that it eliminates the function calling overhead of a traditional function.
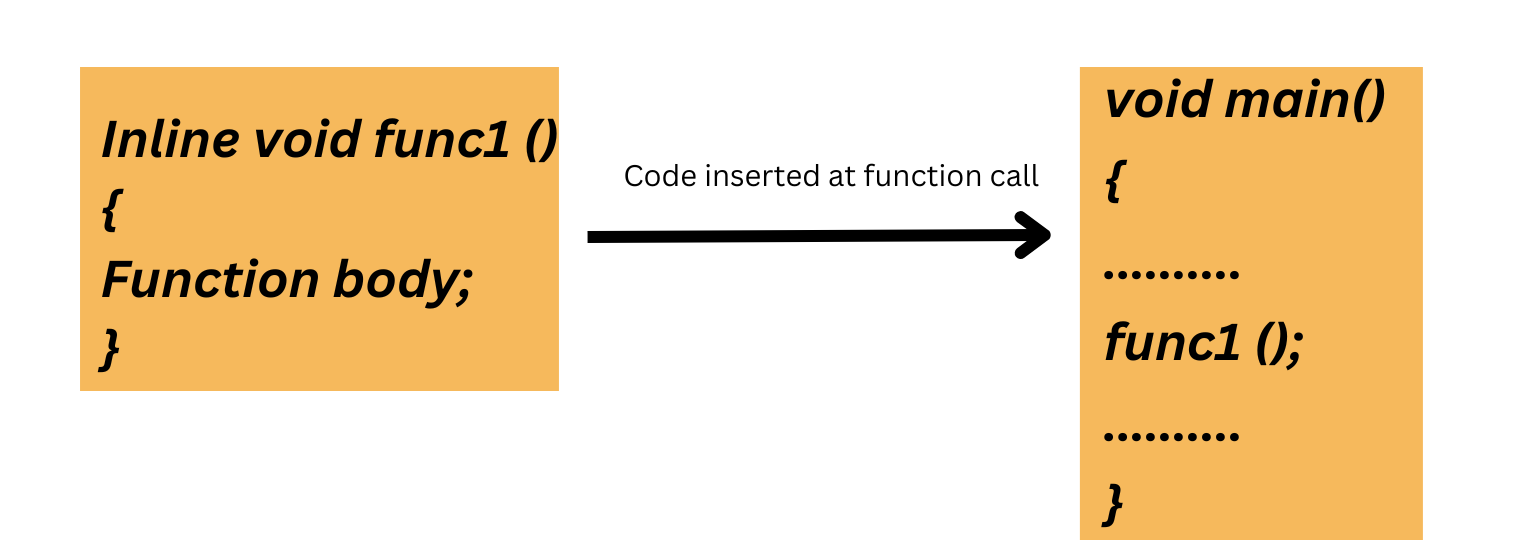
13. What is a scope resolution operator?
A scope resolution operator is represented as :
• This operator is used to associate function definition to a particular class.
• The scope operator is used for the following purposes:
• To access a global variable when you have a local variable with the same name.
• To define a function outside the class.
14. Comment on Local and Global scope of a variable in C++.
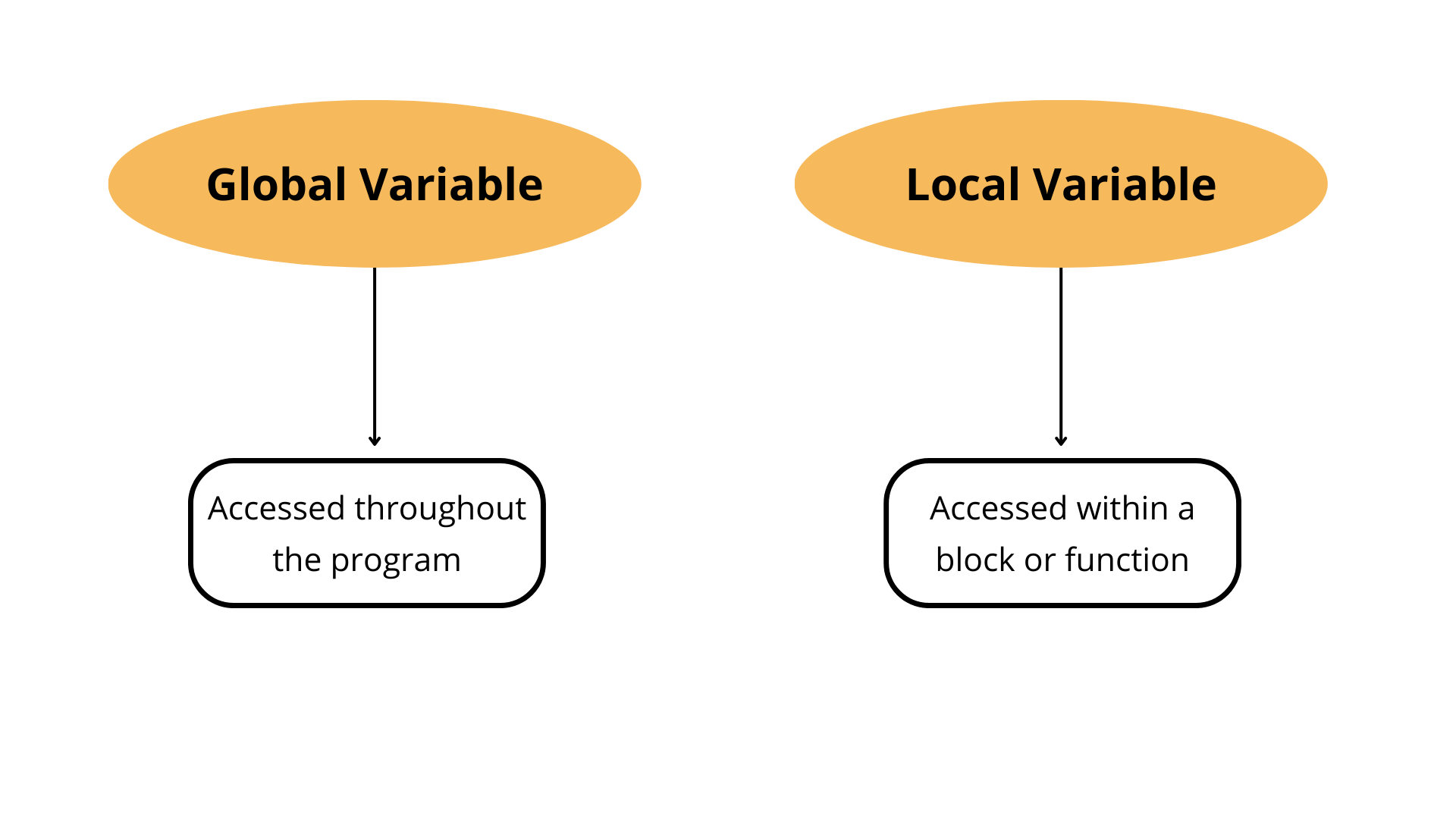
The scope of a variable is defined as the extent of the program code within which the variable remains active i.e. it can be declared, defined or worked with.
There are two types of scope in C++:
Local Scope: A variable is said to have a local scope or is local when it is declared inside a code block. The variable remains active only inside the block and is
not accessible outside the code block.
Global Scope: A variable has a global scope when it is accessible throughout the program. A global variable is declared on top of the program before all the function definitions.
#include <iostream.h> int globalResult=0; //global variable int main() { int localVar = 10; //local variable. }
15. When there are a Global variable and Local variable with the same name, how will you access the global variable?
When there are two variables with the same name but different scope, i.e. one is a local variable and the other is a global variable, the compiler will give preference to a local variable.
In order to access the global variable, we make use of a “scope resolution operator (::)”. Using this operator, we can access the value of the global variable.
#include<iostream.h> int x= 10; int main() { int x= 2; cout<<"Global Variable x = "<<::x; cout<<"\nlocal Variable x= "<<x; }
Output:
Global Variable x = 10
local Variable x= 2
16. How many ways are there to initialize an int with a Constant?
There are two ways:
• The first format uses traditional C notation.
int result = 10;
• The second format uses the constructor notation.
int result (10);
17. How do you define/declare constants in C++?
In C++, we can define our own constants using the #define preprocessor directive.
#include<iostream.h> #define PI 3.142 int main () { float radius =5, area; area = PI * r * r; cout<<"Area of a Circle = "<<area; } Output: Area of a Circle = 78.55
As shown in the above example, once we define a constant using #define directive, we can use it throughout the program and substitute its value.
We can declare constants in C++ using the "const" keyword. This way is similar to that of declaring a variable, but with a const prefix.
Examples of declaring a constant const int pi = 3.142; const char c = "sth"; const zipcode = 411014;
18. What is the difference between equal to (==) and Assignment Operator (=)?
In C++, equal to (==) and assignment operator (=) are two completely different operators.Equal to (==) is an equality relational operator that evaluates two expressions
to see if they are equal and returns true if they are equal and false if they are not. The assignment operator (=) is used to assign a value to a variable. Hence,
we can have a complex assignment operation inside the equality relational operator for evaluation
19. What are the Extraction and Insertion operators in C++? Explain with examples.
In the iostream.h library of C++, cin, and cout are the two data streams that are used for input and output respectively. Cout is normally directed to the screen and cin is assigned to the keyboard.
“cin” (extraction operator): By using overloaded operator >> with cin stream, C++ handles the standard input.
int age;
cin>>age;
As shown in the above example, an integer variable ‘age’ is declared and then it waits for cin (keyboard) to enter the data. “cin” processes the input only when the RETURN key is pressed.
“cout” (insertion operator): This is used in conjunction with the overloaded << operator. It directs the data that followed it into the cout stream.
Example:
cout<<”Hello, World!”;
cout<<123;
20. State the difference between delete and delete[].
“delete[]” is used to release the memory allocated to an array which was allocated using new[]. “delete” is used to release one chunk of memory which was allocated using new.
21. What is a Storage Class? Mention the Storage Classes in C++.
Storage class determines the life or scope of symbols such as variable or functions.
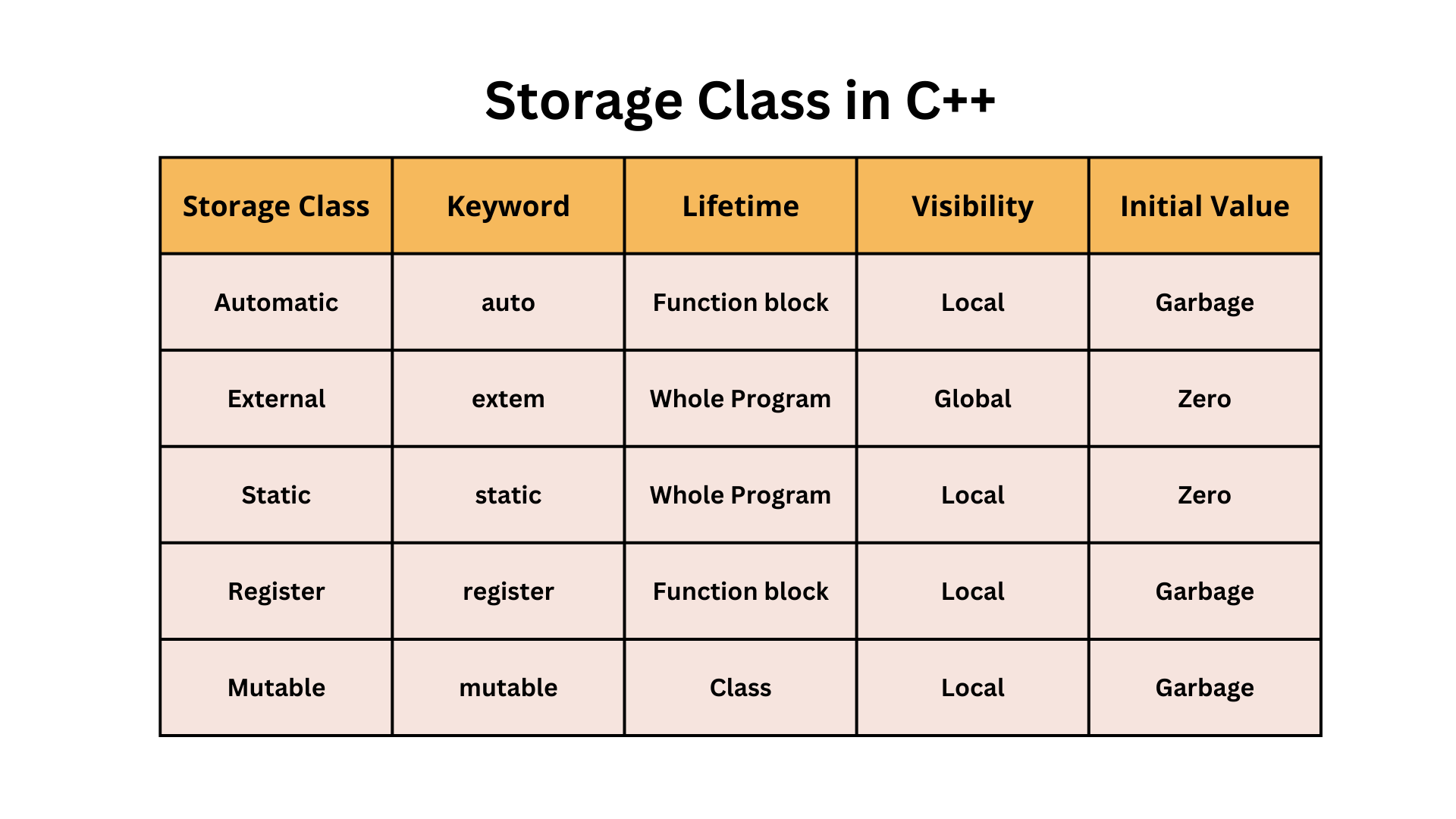
22. What is the difference between a++ and ++a?
• a++ refers to post-increment operation. The C++ compiler first uses the value of a and then increments it.
• ++a refers to the pre-increment operation. The C++ compiler first increments the value of a++ and then uses a.
23. Explain storage qualifiers in C++.
Const: This variable means that if the memory is initialised once, it should not be altered by a program.
Volatile: This variable means that the value in the memory location can be altered even though nothing in the program code modifies the contents.
Mutable: This variable means that a particular member of a structure or class can be altered even if a particular structure variable, class or class member function is constant.
24. Explain dangling pointer.
When the address of an object is used after its lifetime is over, dangling pointer comes into existence.
Some examples of such situations are : Returning the addresses of the automatic variables from a function or using the address of the memory block after it is freed.
25. What is the keyword auto for?
By default, every local variable of the function is automatic i.e. auto. In the below function both the variables ‘i’ and ‘j’ are automatic variables.
void func(){ int i; auto int j; }
💡 A global variable is not an automatic variable.
26. What is the purpose of the Extern Storage Specifier?
“Extern” specifier is used to resolve the scope of a global symbol.
#include <iostream > using nam espace std; main(){ extern int i; cout<<i<<endl; } int i = 20;
In the above code, “i” can be visible outside the file where it is defined.
27. What is stl in C++?
Stl is the standard template library. It is a library that allows you to use a standard set of templates for things such as: Algorithms, functions, Iterators in place of actual code.
28. What is stream in C++?
Stream refers to a stream of characters to be transferred between program thread and i/o.
29. What is enum in C++?
enum is abbreviation of Enumeration which assigns names to integer constant to make a program easy to read. Syntax for the same:
enum enum_name{const1, const2, ……. };
30. What are void pointers?
A void pointer is a pointer which is having no datatype associated with it. It can hold addresses of any type.
For example-
void *ptr; char *str; p=str; // no error str=p; // error because of type mismatch
We can assign a pointer of any type to a void pointer but the reverse is not true unless you typecast it as
str=(char*) ptr;
31. How do you allocate and deallocate memory in C++?
The new operator is used for memory allocation and deletes operator is used for memory deallocation in C++.
For example-
int value=new int; //allocates memory for storing 1 integer delete value; // deallocates memory taken by value int *arr=new int[10]; //allocates memory for storing 10 int delete []arr; // deallocates memory occupied by arr
32. what is a class in C++?
A class in C++ can be defined as a collection of function and related data under a single name. It is a blueprint of objects. A C++ program can consist of any number of classes.
33. How can you specify a class in C++?
By using the keyword class followed by identifier (name of class) you can specify the class in C++. Inside curly brackets, body of the class is defined. It is terminated by semi-colon in the end.
For example, class name{ // some data // some functions };
34. Explain what is the use of void main () in C++ language?
To run the C++ application it involves two steps, the first step is a compilation where conversion of C++ code to object code take place. While second step includes linking, where combining of object code from the programmer and from libraries takes place. This function is operated by main () in C++ language.
35. what is C++ objects?
Class gives blueprints for object, so basically an object is created from a class or in other words an object is an instance of a class. The data and functions are bundled together as a self-contained unit called an object. Here, in the example A and B is the Object.
Class Student { Public: int rollno; String name; } A, B;
36. what is Member Functions in Classes?
The member function regulates the behaviour of the class. It provides a definition for supporting various operations on data held in the form of an object.
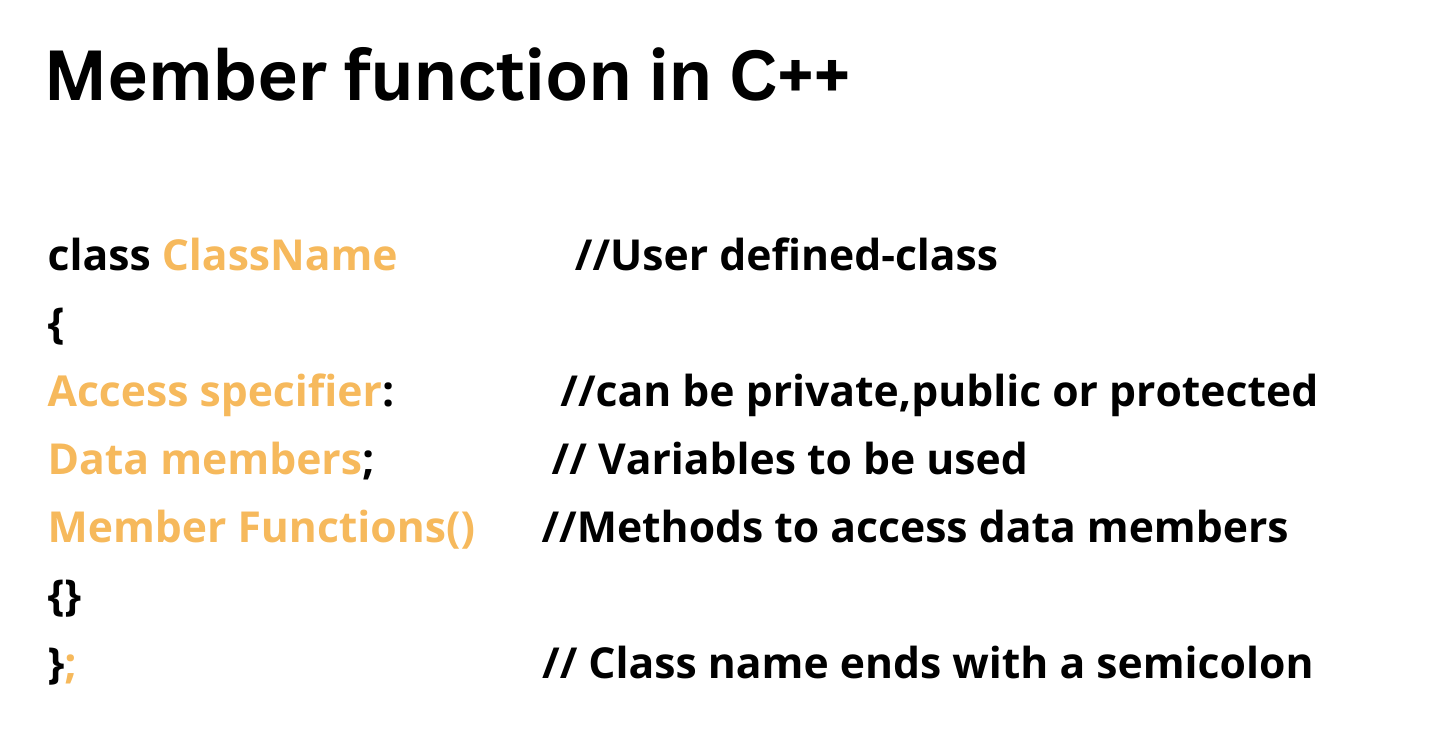
37. What is the difference between an Object and a Class?
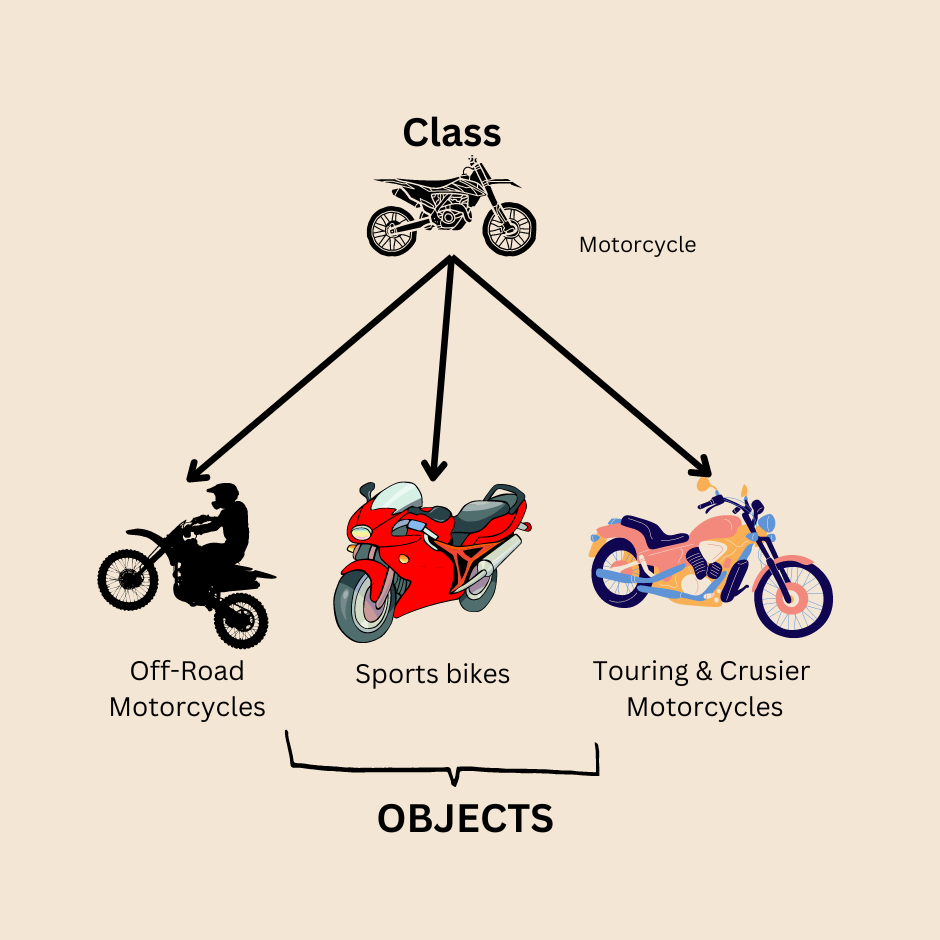
Class is a blueprint of a project or problem to be solved and consists of variables and methods. These are called the members of the class. We cannot access methods or variables of the class on its own unless they are declared static.
In order to access the class members and put them to use, we should create an instance of a class which is called an Object. The class has an unlimited lifetime whereas an object has a limited lifespan only.
38. What are the various Access Specifiers in C++?
C++ supports the following access specifiers:
Public: Data members and functions are accessible outside the class.
Private: Data members and functions are not accessible outside the class. The exception is the usage of a friend class.
Protected: Data members and functions are accessible only to the derived classes.
Example:
Describe PRIVAE, PROTECTED and PUBLIC along with their differences and give examples.
class A{ int x; int y; public int a; protected bool flag; public A() : x(0) , y(0) {} //default (no argument) constructor }; main(){ A MyObj; MyObj.x = 5; // Compiler will issue a ERROR as x is private int x = MyObj.x; // Compiler will issue a compile ERROR MyObj.x is private MyObj.a = 10; // no problem; a is public member int col = MyObj.a; // no problem MyObj.flag = true; // Compiler will issue a ERROR; protected values are read only bool isFlag = MyObj.flag; // no problem
39. What is a Constructor and how is it called?
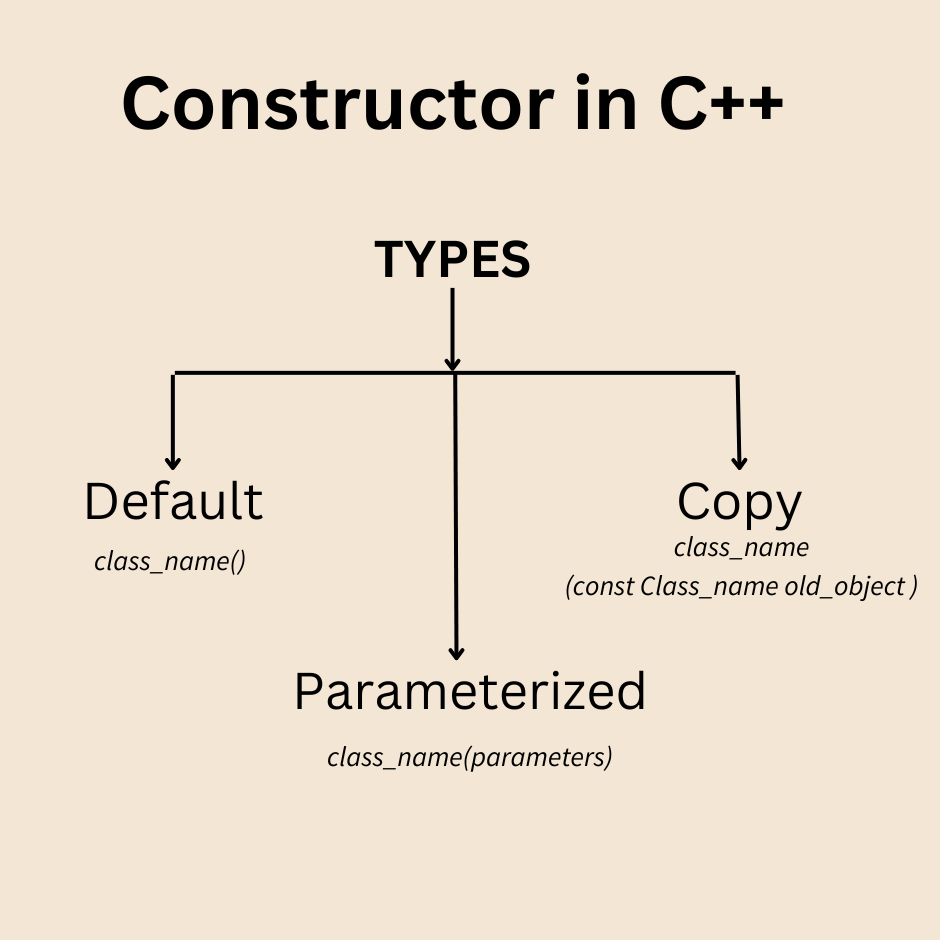
Constructor is a member function of the class having the same name as the class. It is mainly used for initializing the members of the class. By default constructors are public.
There are two ways in which the constructors are called:
Implicitly: Constructors are implicitly called by the compiler when an object of the class is created. This creates an object on a Stack.
Explicit Calling: When the object of a class is created using new, constructors are called explicitly. This usually creates an object on a Heap.
class A{ int x; int y; public A() : x(0) , y(0) {} //default (no argument) constructor }; main() { A Myobj; // Implicit Constructor call. In order to allocate memory on stack, //the default constructor is implicitly called. A * pPoint = new A(); // Explicit Constructor call. In order to allocate //memory on HEAP we call the default constructor. }
40. What is a COPY CONSTRUCTOR and when is it called?
A copy constructor is a constructor that accepts an object of the same class as its parameter and copies its data members to the object on the left part of the assignment. It is useful when we need to construct a new object of the same class.
class A{ int x; int y; public int color; public A() : x(0) , y(0) {} //default (no argument) constructor public A( const A& ) ; }; A::A( const A & p ) { this->x = p.x; this->y = p.y; this->color = p.color; } main() { A Myobj; Myobj.color = 345; A Anotherobj = A( Myobj ); // now Anotherobj has color = 345 }
41. What is a Default Constructor?
Default constructor is a constructor that either has no arguments or if there are any, then all of them are default arguments.
Example: class B { public: B (int m = 0) : n (m) {} int n; }; int main(int argc, char *argv[]) { B b; return 0; }
42. What is a Conversion Constructor?
It is a constructor that accepts one argument of a different type. Conversion constructors are mainly used for converting from one type to another.
43. What is operator overloading?
Operator Overloading is a very essential element to perform the operations on user-defined data types. By operator overloading we can modify the default meaning to the operators like +, -, *, /, <=, etc.
For example -
The following code is for adding two complex number using operator overloading-
class complex{ private: float r, i; public: complex(float r, float i){ this->r=r; this->i=i; } complex(){} void displaydata(){ cout<<"real part = "<<r<<endl; cout<<"imaginary part = "<<i<<endl; } complex operator+(complex c){ return complex(r+c.r, i+c.i); } }; int main(){ complex a(2,3); complex b(3,4); complex c=a+b; c.displaydata(); return 0; }
44. What is the role of the Static keyword for a class member variable?
The static member variable shares a common memory across all the objects created for the respective class. We need not refer to the static member variable using an object. However, it can be accessed using the class name itself.
45. What’s the order in which the local objects are destructed?
Consider following a piece of code:
Class A{ ... }; int main() { A a; A b; ... }
In the main function, we have two objects created one after the other. They are created in order, first a then b. But when these objects are deleted or if they go out of the scope, the destructor for each will be called in the reverse order in which they were constructed. Hence, the destructor of b will be called first followed by a. Even if we have an array of objects, they will be destructed in the same way in the reverse order of their creation.
46. What is the difference between Method Overloading and Method Overriding in C++?
Method overloading is having functions with the same name but different argument lists. This is a form of compile-time polymorphism.
Method overriding comes into picture when we rewrite the method that is derived from a base class. Method overriding is used while dealing with run-time polymorphism or virtual functions.
47. Name the Operators that cannot be Overloaded.
sizeof – sizeof operator
. – Dot operator
.* – dereferencing operator
-> – member dereferencing operator
:: – scope resolution operator
?: – conditional operator
48. Function can be overloaded based on the parameter which is a value or a reference. Explain if the statement is true.
False. Both, Passing by value and Passing by reference look identical to the caller.
49. What is Inheritance?
Inheritance is a process by which we can acquire the characteristics of an existing entity and form a new entity by adding more features to it. In terms of C++, inheritance is creating a new class by deriving it from an existing class so that this new class has the properties of its parent class as well as its own.
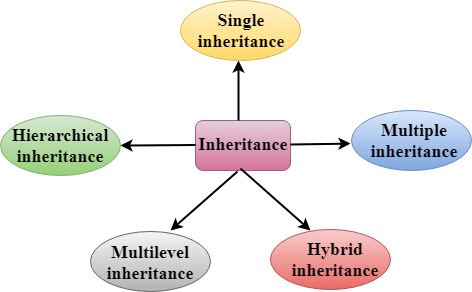
50. Does C++ support Multilevel and Multiple Inheritances?
Yes
51. Does a derived class inherit or doesn’t inherit?
When a derived class is constructed from a particular base class, it basically inherits all the features and ordinary members of the base class. But there are some exceptions to this rule. For instance, a derived class does not inherit the base class’s constructors and destructors.
Each class has its own constructors and destructors. The derived class also does not inherit the assignment operator of the base class and friends of the class. The reason is that these entities are specific to a particular class and if another class is derived or if it is the friend of that class, then they cannot be passed onto them.
53. What are Multiple Inheritances (virtual inheritance)? What are its advantages and disadvantages?
In multiple inheritances, we have more than one base classes from which a derived class can inherit. Hence, a derived class takes the features and properties of more than one base class.
For Example, a class driver will have two base classes namely, employee and a person because a driver is an employee as well as a person. This is advantageous because the driver class can inherit the properties of the employee as well as the person class.
But in the case of an employee and a person, the class will have some properties in common. However, an ambiguous situation will arise as the driver class will not know the classes from which the common properties should be inherited. This is the major disadvantage of multiple inheritances.
52. Explain the ISA and HASA class relationships. How would you implement each?
“ISA” relationship usually exhibits inheritance as it implies that a class “ISA” specialized version of another class. For Example, An employee ISA person. That means an Employee class is inherited from the Person class.
Contrary to “ISA”, “HASA” relationship depicts that an entity may have another entity as its member or a class has another object embedded inside it.
So taking the same example of an Employee class, the way in which we associate the Salary class with the employee is not by inheriting it but by including or containing the Salary object inside the Employee class. “HASA” relationship is best exhibited by containment or aggregation.
54. What is polymorphism in C++?
Polymorphism in simple means having many forms. Its behavior is different in different situations. And this occurs when we have multiple classes that are related to each other by inheritance. For example, think of a base class called a car that has a method called car brand(). Derived classes of cars could be Mercedes, BMW, Audi - And they also have their own implementation of a cars
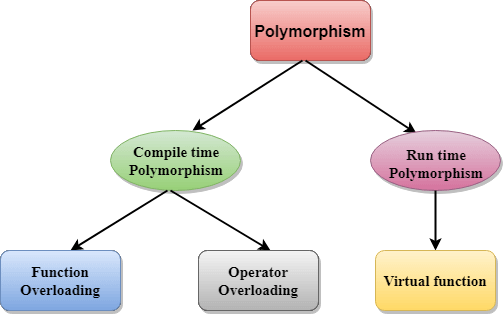
The two types of polymorphism in c++ are:
• Compile Time Polymorphism
• Runtime Polymorphism
55. What do you know about friend class and friend function?
A friend class can access private, protected, and public members of other classes in which it is declared as friends. Like friend class, friend function can also access private,
protected, and public members. But, Friend functions are not member functions.
For example -
class A{ private: int data_a; public: A(int x){ data_a=x; } friend int fun(A, B); } class B{ private: int data_b; public: A(int x){ data_b=x; } friend int fun(A, B); } int fun(A a, B b){ return a.data_a+b.data_b; } int main(){ A a(10); B b(20); cout<<fun(a,b)<<endl; return 0; }
56. What is Name Mangling?
C++ compiler encodes the parameter types with function/method into a unique name. This process is called name mangling. The inverse process is called as demangling.
Example:
A::b(int, long) const is mangled as ‘b__C3Ail’.
For a constructor, the method name is left out.
That is A:: A(int, long) const is mangled as ‘C3Ail’.
57. What are Virtual Functions?
A virtual function allows the derived classes to replace the implementation provided by the base class. Whenever we have functions with the same name in the base as well as derived class, there arises an ambiguity when we try to access the child class object using a base class pointer. As we are using a base class pointer, the function that is called is the base class function with the same name.
To correct this ambiguity we use the keyword “virtual” before the function prototype in the base class. In other words, we make this polymorphic function Virtual. By using a Virtual function, we can remove the ambiguity and we can access all the child class functions correctly using a base class pointer.
58. What do you mean by Pure Virtual Functions?
A Pure Virtual Member Function is a member function in which the base class forces the derived classes to override. Normally this member function has no implementation. Pure virtual functions are equated to zero.
Example:
class Shape { public: virtual void draw() = 0; };
Base class that has a pure virtual function as its member can be termed as an “Abstract class”. This class cannot be instantiated and it usually acts as a blueprint that has several sub-classes with further implementation.
59. What is a friend function?
C++ class does not allow its private and protected members to be accessed outside the class. But this rule can be violated by making use of the “Friend” function.
As the name itself suggests, friend function is an external function that is a friend of the class. For friend function to access the private and protected methods of the class, we should have a prototype of the friend function with the keyword “friend” included inside the class.
60. What is a friend class?
Friend classes are used when we need to override the rule for private and protected access specifiers so that two classes can work closely with each other.
Hence, we can have a friend class to be a friend of another class. This way, friend classes can keep private, inaccessible things in the way they are.
When we have a requirement to access the internal implementation of a class (private member) without exposing the details by making the public, we go for friend functions.
60. What is Exception Handling? Does C++ support Exception Handling?
Yes C++ supports exception handling. We cannot ensure that code will execute normally at all times. There can be certain situations that might force the code written by us to malfunction, even though it’s error-free. This malfunctioning of code is called Exception. When an exception has occurred, the compiler has to throw it so that we know an exception has occurred. When an exception has been thrown, the compiler has to ensure that it is handled properly, so that the program flow continues or terminates properly. This is called the handling of an exception. Thus in C++, we have three keywords i.e. try, throw and catch which are in exception handling.
61. What is an Iterator class?
In C++ a container class is a collection of different objects. If we need to traverse through this collection of objects, we cannot do it using simple index variables. Hence, we have a special class in STL called an Iterator class which can be used to step through the contents of the container class.
The various categories of iterators include input iterators, output iterators, forward iterators, bidirectional iterators, random access, etc.
62. What is the difference between an External Iterator and an Internal Iterator? Describe an advantage of the External Iterator.
An internal iterator is implemented with member functions of the class that has items to step through.
An external iterator is implemented as a separate class that can be bound to the object that has items to step through. The basic advantage of an External iterator is that it’s easy to implement as it is implemented as a separate class.
Secondly, as it’s a different class, many iterator objects can be active simultaneously.
63. What is vector in C++?
A sequence of containers to store elements, a vector is a template class of C++. Vectors are used when managing ever-changing data elements. The syntax of creating vector.
vector (type) variable (number of elements)
64. How to use map in C++?
Associative containers storing a combination of a key value or mapped value is called Maps.
Syntax: map<key_type , value_type> map_name; #include <iostream> #include <iterator> #include <map> using namespace std; int main() { map<int, int> test; // inserting elements test.insert(pair<int, int>(1, 2)); test.insert(pair<int, int>(2, 3)); map<int, int>::iterator itr; for (itr = test.begin(); itr != test.end(); ++itr) { cout << itr->first cout << itr->second << '\n'; } return 0; )
65. What is namespace std; and what is consists of?
Namespace std; defines your standard C++ library, it consists of classes, objects and functions of the standard C++ library. You can specify the library by using namespace std or std: : throughout the code. Namespace is used to differentiate the same functions in a library by defining the name.
66. Explain what is upcasting in C++?
Upcasting is the act of converting a sub class references or pointer into its super class reference or pointer is called upcasting.
67. Explain what is pre-processor in C++?
Pre-processors are the directives, which give instruction to the compiler to pre-process the information before actual compilation starts.
68. Define token in C++.
A token in C++ can be a keyword, identifier, literal, constant and symbol.
69. What is function overriding?
If you inherit a class into a derived class and provide a definition for one of the base class's function again inside the derived class, then this function is called overridden function, and this mechanism is known as function overriding.
70. What is a pure virtual function?
The pure virtual function is a virtual function which does not contain any definition. The normal function is preceded with a keyword virtual. The pure virtual function ends with 0.
Syntax of a pure virtual function:
virtual void abc()=0; //pure virtual function.
71. What is the difference between function overloading and operator overloading?
Function overloading: Function overloading is defined as we can have more than one version of the same function. The versions of a function will have different signature means that they have a different set of parameters.
Operator overloading: Operator overloading is defined as the standard operator can be redefined so that it has a different meaning when applied to the instances of a class.
72. What is a virtual destructor?
A virtual destructor in C++ is used in the base class so that the derived class object can also be destroyed. A virtual destructor is declared by using the ~ tilde operator and then virtual keyword before the constructor.
💡 Constructor cannot be virtual, but destructor can be virtual.
73. If class D is derived from a base class B. When creating an object of type D in what order would the constructors of these classes get called?
The derived class has two parts, a base part, and a derived part. When C++ constructs derived objects, it does so in phases. First, the most-base class(at the top of the inheritance tree) is constructed. Then each child class is constructed in order until the most-child class is constructed last. So the first Constructor of class B will be called and then the constructor of class D will be called.
During the destruction exactly reverse order is followed. That is destructor starts at the most-derived class and works its way down to base class. So the first destructor of class D will be called and then the destructor of class B will be called.
74. Can we call a virtual function from a constructor?
Yes, we can call a virtual function from a constructor. But the behavior is a little different in this case. When a virtual function is called, the virtual call is resolved at runtime. It is always the member function of the current class that gets called. That is the virtual machine doesn’t work within the constructor.
For Example:
class base{ private: int value; public: base(int x){ value=x; } virtual void fun(){ } } class derived{ private: int a; public: derived(int x, int y):base(x){ base *b; b=this; b->fun(); //calls derived::fun() } void fun(){ cout<<"fun inside derived class"<<endl; } }
75. What do you mean by ‘void’ return type?
All functions should return a value as per the general syntax. However, in case, if we don’t want a function to return any value, we use “void” to indicate that. This means that we use “void” to indicate that the function has no return value or it returns “void”.
Example: void myfunc() { cout <<"Hello,This is my function!!"; } int main() { myfunc(); return 0; }
76. Explain Pass by Value and Pass by Reference.
While passing parameters to the function using “Pass by Value”, we pass a copy of the parameters to the function.
Hence, whatever modifications are made to the parameters in the called function are not passed back to the calling function. Thus the variables in the calling function remain unchanged.
void printFunc(int a,int b,int c) { a *=2; b *=2; c *=2; } int main() { int x = 1,y=3,z=4; printFunc(x,y,z); cout<<"x = "<<x<<"\ny = "<<y<<"\nz = "<<z; }
As seen above, although the parameters were changed in the called function, their values were not reflected in the calling function as they were passed by value. However, if we want to get the changed values from the function back to the calling function, then we use the “Pass by Reference” technique.
To demonstrate this we modify the above program as follows:
void printFunc(int& a,int& b,int& c) { a *=2; b *=2; c *=2; } int main() { int x = 1,y=3,z=4; printFunc(x,y,z); cout<<"x = "<<x<<"\ny = "<<y<<"\nz = "<<z; }
77. What are Default Parameters? How are they evaluated in the C++ function?
Default Parameter is a value that is assigned to each parameter while declaring a function. This value is used if that parameter is left blank while calling to the function. To specify a default value for a particular parameter, we simply assign a value to the parameter in the function declaration.
If the value is not passed for this parameter during the function call, then the compiler uses the default value provided. If a value is specified, then this default value is stepped on and the passed value is used.
int multiply(int a, int b=2) { int r; r = a * b; return r; } int main() { cout<<multiply(6); cout<<"\n"; cout<<multiply(2,3); }
As shown in the above code, there are two calls to multiply function. In the first call, only one parameter is passed with a value. In this case, the second parameter is the default value provided. But in the second call, as both the parameter values are passed, the default value is overridden and the passed value is used.
78. Why are arrays usually processed with for loop?
Array uses the index to traverse each of its elements.
If A is an array then each of its elements is accessed as A[i]. Programmatically, all that is required for this to work is an iterative block with a loop variable i that serves as an index (counter) incrementing from 0 to A.length-1. This is exactly what a loop does and this is the reason why we process arrays using for loops.
79. What is wrong with this code?
T *p = new T[10];
delete p;
The above code is syntactically correct and will compile fine.
The only problem is that it will just delete the first element of the array. Though the entire array is deleted, only the destructor of the first element will be called and the memory for the first element is released.
79. What is wrong with this code?
T *p = 0;
delete p;
In the above code, the pointer is a null pointer. Per the C++ 03 standard, it’s perfectly valid to call delete on a NULL pointer. The delete operator would take care of the NULL check internally.
80. What should be the correct statement about string objects in C++?
1. String objects should necessarily be terminated by a null character
2. String objects have a static size
3. String objects have a dynamic size
4. String objects use extra memory than required
5. String objects have a dynamic size.
81. What is Abstraction?
Abstraction can be defined as a technique in which you only show functionality to the user i.e., the details that you want the user to see, hiding the internal details or implementation details.
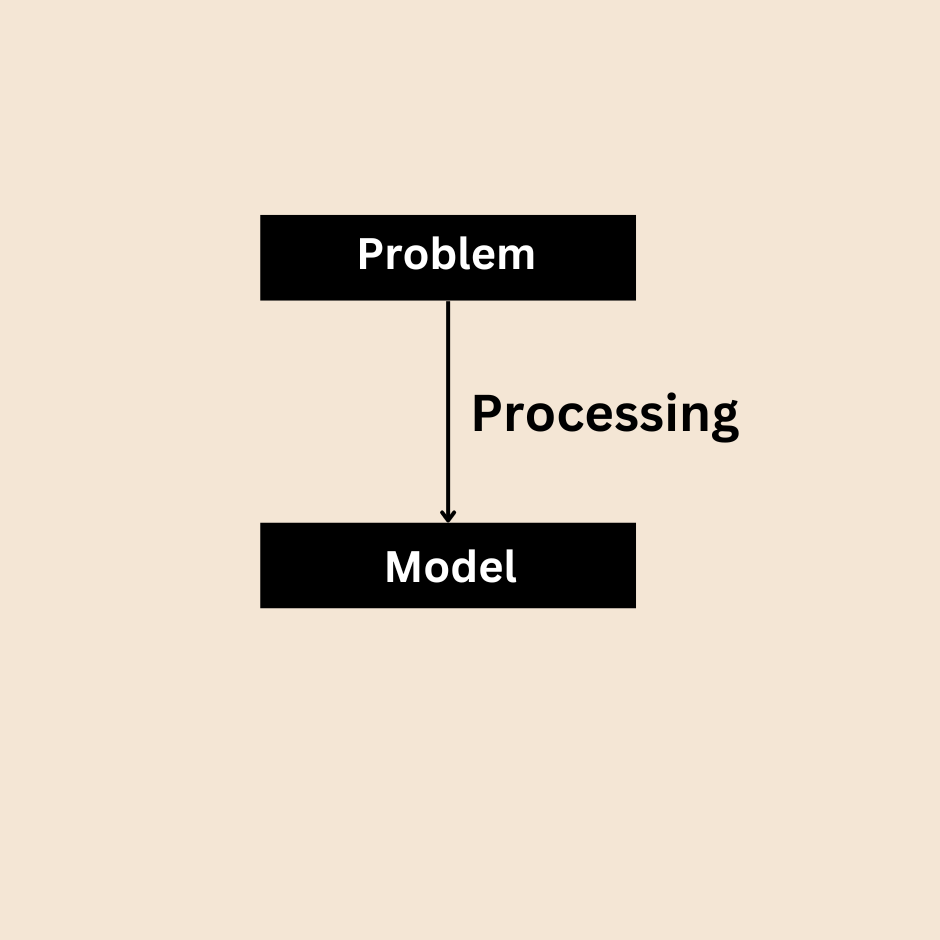
82. How would you establish the relationship between abstraction and encapsulation?
Data abstraction refers to representing only the important features of the real-world object in the C++ program. This entire process does not include any explanation and background details of the code. Abstraction is implemented with the help of classes and objects, whereas, data encapsulation is the most significant feature of a class.
Data encapsulation in C++ refers to the wrapping up of data members and member functions that operate on the data as a single unit, called the class. Encapsulation prevents free access to the members of the class.
83. How to reverse a string in C++?
To reverse a string, a sample code is mentioned below.
#include<iostream> #include<string.h> using namespace std; int main () { char n[50], t; int i, j; cout << "Enter a string : "; gets(n); i = strlen(n) - 1; for (j = 0; j < i; j++,i--) { t = s[j]; s[j] = s[i]; s[i] = t; } cout << "\nReverse string : " << s; return 0; }
🚀Conclusion
Finally, this page has come to a close. You should be able to build your own programmes with the material on this page and little study, and small projects are actually encouraged for improving your programming skills. There's no way to cover everything you need to know about programming in a single class. Programming is a never-ending learning process, whether you're a seasoned professional developer or a total beginner.
Frequently Asked Questions (FAQs)
• A key idea in object-oriented programming (OOP), polymorphism enables objects to display a variety of behaviors or take on many forms. By considering objects of several classes as belonging to a single base class, it promotes code reuse, flexibility, and extension. In C++, polymorphism is made possible through tools like virtual functions and function overriding.
• Base class and derived class are two crucial concepts to comprehend while discussing polymorphism. A base class is a class that specifies shared traits and actions among several derived classes. A derived class, on the other hand, is a class that both adds its own special characteristics while also inheriting properties and behaviors from the base class.
• Polymorphism in C++ is commonly accomplished via virtual functions. A member function that is declared in a base class and can be overridden by a derived class is known as a virtual function. A virtual function's specific implementation in the derived class is invoked when it is called using a pointer or reference to the base class.
• The virtual function must be declared in the base class using the 'virtual' keyword in order to enable polymorphism. The 'override' keyword is used by a derived class to indicate that it is purposefully overriding the implementation of the base class when it calls a virtual function. This makes sure that at runtime, the appropriate derived class function is invoked.
• High levels of flexibility in program design and implementation are made possible through polymorphism. It makes it easier to write code that can interact with objects of many classes, treating them consistently based on their common base class. As a result, there is less need for duplicate code, which encourages code reuse and streamlines maintenance.
• The use of dynamic binding or late binding is one of the primary advantages of polymorphism. The right function implementation can be chosen at runtime based on the actual type of the object being referenced thanks to dynamic binding. Static binding, on the other hand, resolves the function implementation at compile time.
• Function overloading is a different type of polymorphism that is supported by C++ in addition to virtual functions. Function overloading enables the coexistence of many functions in a class that share the same name but have different argument lists. The number, kind, and order of the parameters provided to a function decide which one should be invoked. By employing function overloading, several operations can be carried out on various data types while still using the same function name.
• To put it simply, polymorphism in C++ refers to an object's capacity to display a variety of behaviors or adopt various shapes. It is made possible by features like virtual functions and function overriding, which let objects of various classes be treated as though they were members of the same base class. Polymorphism is a key idea in object-oriented programming because it encourages code reuse, flexibility, and extensibility.
1. Polymorphism allows items to display a variety of behaviors or take on several forms.
2. Function overriding and virtual functions are used to do it.
3. In object-oriented programming, polymorphism encourages code reuse, adaptability, and extension.
The capabilities of the C programming language are expanded by the general-purpose programming language C++. Combining procedural programming and object-oriented programming (OOP) elements is how it is intended to work. Classes, objects, inheritance, and polymorphism are some of C++'s fundamental building blocks.
1. Classes
The idea of classes is at the heart of C++. A class is a sort of data that the user defines that combines information (attributes) and operations (methods) into a single unit. It acts as a guide or model for building things. Classes enable programmers to produce reusable and modular code by defining the structure and behavior of objects.
2. Objects:
Classes have instances that are objects. A class instantiates itself by producing an object in memory. Objects have behavior (methods that operate on the data) and state (data kept in member variables). They can conduct acts, communicate, and interact with other objects. Real-world entities can be modeled using the concept of objects, which also makes it possible to organize and encapsulate code.
3. Inheritance
The method of inheritance in C++ enables the construction of new classes (derived classes) from base classes. Code reuse and hierarchical relationships are encouraged by the fact that derived classes inherit the traits and characteristics of their base classes. Because of inheritance, it is possible to build specialized classes that add to and extend the capabilities of their underlying classes. It makes it easier to understand the idea of "is-a" relationships, in which a derived class is a particular kind of the base class.
4. polymorphism
A key idea in object-oriented programming enabled by C++ is polymorphism. It describes an object's capacity to assume many shapes or exhibit a variety of behaviors. Features like virtual functions and function overriding enable polymorphism. It promotes the flexibility, extensibility, and reuse of code by enabling the treatment of objects of several classes as if they were objects of a single base class.
5. Additional Ideas:
Encapsulation, data abstraction, templates, and exception handling are among the extra concepts included in C++. Encapsulation is the controlled access to data and methods that are combined within a class. Data abstraction involves simplification of complex data processes and types. Generic programming is made possible through templates, which produce reusable code that can handle many data types. Programs can handle errors and recover gracefully thanks to exception handling.
Programmers can create effective, modular, and reusable code using C++'s comprehensive feature set and syntax. System software, game development, embedded devices, and high-performance applications are just a few of the many applications that make use of it. C++ gives developers flexibility and ability to build reliable and scalable software solutions by fusing the procedural programming paradigm with object-oriented ideas.
Key facts:
1. The C++ programming language expands the capabilities of the C programming language.
2. Classes, objects, inheritance, and polymorphism are the core ideas of C++.
3. Classes act as templates for building objects, which are containers for data and action.
4. Code reuse and class hierarchy are both made possible via inheritance.
5. Polymorphism makes it possible to treat objects of several classes as if they were members of a single base class.
• As an expansion of the C programming language, C++ is a general-purpose computer language. It was developed to keep C compatible while offering more features and functionalities. The procedural programming model of C is combined with potent object-oriented programming (OOP) features in C++. In fields including system software, gaming development, embedded systems, and high-performance applications, it is frequently utilized to create a wide range of software applications.
• Bjarne Stroustrup created C++ for the first time in the late 1970s under the name "C with Classes." Over time, the language changed, adding new functions and improvements. C++ programmers can migrate to C++ quite easily because it keeps the syntax and many features of the C language. However, it also provides new ideas and procedures that greatly increase C's capabilities.
• Support for object-oriented programming is one of C++'s core characteristics. Classes can be created in C++ and act as a guide for building objects. Data (attributes) and functions (methods) are combined into a single object known as a class. Programmers can now organize their code in a modular and reusable way. Real-world entities can be modeled using objects, which are instances of classes, because they have their own state (data) and behavior (methods), as well as the ability to communicate with one another.
• Object-oriented programming is only one of the strong aspects that C++ offers. Through the use of templates, it facilitates generic programming and enables the development of generic classes and functions that can operate on many data types. Templates encourage code reuse and make it possible to create extremely flexible and effective algorithms. Additionally, C++ has features like operator overloading, support for low-level programming, and exception handling that make it appropriate for a variety of applications.
• Due to its adaptability, C++ is used extensively across a variety of industries. Due to its low-level capabilities and effectiveness, C++ is frequently used in system software development for operating systems, compilers, and device drivers. Because of C++'s performance needs and capacity for handling intricate simulations and graphics, game creation strongly depends on it. The effective memory management and hardware control characteristics of C++ are advantageous for embedded systems like microcontrollers and Internet of Things devices. Applications that require high performance, such simulations and scientific computing, make use of C++'s capacity to speed up and streamline code.
• To summarize, the C language's capabilities are increased by the powerful and popular programming language C++. It enables code organization and reuse by fusing procedural programming with object-oriented characteristics. Templates, operator overloading, and exception handling are some of the extra features that C++ offers, making it suited for a variety of applications in system software, game development, embedded systems, and high-performance computing.
• An object in C++ is a particular instance of a class. It represents a particular object or data structure that was built using the instructions supplied by the class. Every object has a distinct identity, state, and behavior. They combine functions and data into a single unit, enabling effective data organization and manipulation.
• When a class is defined, it acts as a model or blueprint for the characteristics and actions that an object of that class will possess. It specifies the member functions (also known as methods), which describe the behavior or actions that may be carried out on the object, as well as the data members (also known as attributes or properties), which represent the state of the object.
• In C++, you call a function by using parenthesis around the class name to construct an object. Instantiation is the term for this action. Memory is allocated to house the object's data members during instantiation, and the constructor, a unique member function, is called to set the object's state.
• Once an object has been created, it can be used by calling it by name and using the dot operator (.). This activates the object's member functions and grants access to the object's data members. By calling methods on other objects or accessing their characteristics, objects can communicate with one another.
• The core of the object-oriented programming (OOP) paradigm is the idea of objects. Real-world entity modeling and modular, reusable programming are made possible by objects. They enable encapsulation, which ensures data integrity and code structure by enclosing the data and methods associated with an object within the object.
• The idea of inheritance in C++, where classes can take characteristics and actions from other classes, is also influenced by objects. The ability to create specialized classes from pre-existing classes through inheritance encourages code reuse and a hierarchy of class relationships.
• In C++, using objects has a number of advantages. By grouping related data and functions into objects, it enables the building of modular and reusable code. A simple and natural way to represent and communicate with entities in the issue domain is through the use of objects. They make it possible to apply the principles of encapsulation, data hiding, and information concealing, improving the dependability and maintainability of the code.
• In C++, entities or data structures are represented by classes, and objects are instances of those classes. They provide a mechanism to model real-world items and enable the structure and reuse of code by encapsulating data and behaviors. For characteristics like encapsulation and inheritance, objects are essential to the object-oriented programming paradigm.
The syntax, data types, control structures, and functions are the four fundamental parts of C++. The building blocks of the language, these elements are necessary for creating C++ programs.
1. Syntax
The grammar and structure of the C++ language are governed by a set of rules and guidelines, which are referred to as syntax. To write programs that are legitimate and understandable, it specifies how statements and expressions should be constructed. Rules for declaring variables, defining functions, managing program flow, and arranging code using blocks and scopes are all part of the C++ syntax. For the compiler to comprehend and interpret the program correctly, proper syntax must be followed.
2. Data Types
In C++, data types specify the kinds of data that can be stored in variables. They outline the assortment of values variables can hold as well as the activities that can be carried out on them. Fundamental data types like integers, floating-point numbers, characters, and Boolean values are supported by a number of built-in data types in C++. Additionally, it offers more complex data types like pointers, classes, arrays, and structures. Programmers can efficiently allocate memory and handle data thanks to data types.
3. Control Structures
In C++, control structures control how a program is executed. They enable decision-making, repeating activities, and branching based on certain circumstances. Conditional statements (such as if-else and switch statements), which run distinct blocks of code depending on given conditions, are one of the control structures offered by C++. Additionally, it has loop statements (such for, while, and do-while loops) that repeatedly run a series of instructions up until a certain condition is satisfied. Programmers are able to develop dynamic, adaptable programs thanks to control structures.
4. Functions
In C++, functions are reusable sections of code that carry out particular duties. They can accept input parameters and return a value while encapsulating a series of instructions. Functions enable programmers to break down a program into more manageable, smaller components, promoting modularity and code reuse. Both user-defined functions, which programmers write to carry out certain tasks, and built-in functions, such as mathematical functions, are supported by C++. Functions enhance the readability, maintainability, and organization of the code.
Together, these four C++ building blocks make up the language's core. Programmers can create well-organized, effective, and scalable C++ programs by successfully using the syntax, data types, control structures, and functions.
Important details:
1. The syntax, data types, control structures, and functions are C++'s four primary building blocks.
2. Syntax outlines the language's grammatical structure.
3. Data types define the kinds of information that variables can store.
4. Control structures decide how a program will be executed.
5. Reusable code pieces called functions carry out particular duties.
The most challenging aspect of C++ might differ from person to person depending on background, experience, and familiarity with programming ideas. However, many programmers find a few C++ features to be particularly difficult. These consist of:
1. Pointers and Memory Management: In C++, pointers enable direct access to and manipulation of memory addresses. Although they offer strong capabilities, they can be difficult to comprehend and effectively use. To prevent memory leaks or invalid memory access, memory management, including dynamic memory allocation and deallocation using methods like "new" and "delete," requires careful handling. Pointer handling errors can result in flaws like segmentation faults, memory corruption, and other challenging problems.
2. Template Metaprogramming: Generic programming is made possible by C++ templates, allowing the development of reusable code that functions with many data types. This idea is developed further by template metaprogramming, which generates code and performs computations at compile time using templates. It makes use of sophisticated methods like recursive template instantiation, type characteristics, and template specialization. Understanding template syntax, ideas like template deduction and instantiation, and utilizing template features to achieve desired compile-time behaviors make up the complexity of template metaprogramming.
3. Virtual Functions and Multiple Inheritance: C++ offers multiple inheritance, enabling a class to derive from various base classes. Multiple inheritance, albeit effective, can make it difficult to manage the connections between several base classes and deal with potential ambiguities. Concepts like virtual function tables (vtables) and virtual function resolution, which can be challenging for beginners to understand, are introduced when using virtual functions in conjunction with multiple inheritance. It necessitates comprehension of the function call hierarchy and the handling of overridden functions.
4. Standard Template Library (STL): The Standard Template Library (STL) is a set of universally applicable iterators, containers, and algorithms made available by C++. It provides a wide range of functionalities, such as data structures like vectors, lists, and maps, as well as sorting, searching, and data-manipulating algorithms. Although the STL offers developers strong tools, learning it can be difficult due to its wide functionality and complex syntax. It is important to be familiar with the fundamental ideas behind STL containers, iterators, and algorithms in order to use them correctly.
5. Exception Handling: To manage and recover from runtime mistakes, C++ offers exception handling techniques. Try-catch blocks are used in exception handling to catch and deal with errors that happen while a program is running. Understanding exception types, exception propagation, handling exceptions across function calls, and resource cleanup using techniques like RAII (Resource Acquisition Is Initialization) are all necessary for effective exception management. Effective exception handling and preventing memory leaks or other resource problems can be difficult C++ programming tasks.
It's crucial to remember that while these C++ features can be difficult at times, they also offer programming flexibility and strong possibilities. These difficulties can be solved with time, effort, and a thorough knowledge of the language. It can be quite helpful to use tools like documentation, tutorials, and practice exercises to help you learn the challenging areas of C++ programming.
• Yes, being proficient in C++ can be enough to land a job in the programming or software development industry. Particularly in fields like system software, game development, embedded systems, and high-performance computing, C++ is a popular and in-demand programming language.
• In fields where C++ is widely used, proficiency in it opens up a number of work options. Jobs like software engineer, systems programmer, game developer, embedded systems developer, algorithm developer, and performance engineer are just a few examples.
• Due to its effectiveness, adaptability, and widespread use in vital software systems, C++ proficiency is in high demand from employers. Knowledge of memory management, low-level programming ideas, and algorithmic effectiveness are all strongly implied by proficiency in C++.
• It's crucial to remember, too, that employers frequently search for a larger range of abilities and knowledge in addition to C++. Other programming languages, a comprehension of software development processes, familiarity with data structures and algorithms, practical knowledge of software libraries and frameworks, and problem-solving skills are a few examples of what this could entail.
• Complementing your C++ knowledge with expertise in related fields, such as object-oriented programming (OOP) principles, data structures and algorithms, design patterns, version control systems, debugging techniques, and software testing procedures might be beneficial for improving your job prospects.
• Additionally, acquiring real-world experience through internships, side projects, or donations to open-source software can greatly improve your prospects of finding employment. A solid portfolio that illustrates your ability to apply C++ and associated abilities to real-world projects is highly valued by employers. You should also have practical experience.
• In conclusion, while C++ expertise can be enough to land a job, it is advantageous to have a broad skill set and a firm grasp of software development concepts. You may make yourself stand out in the crowded job market and improve your career prospects by regularly updating your knowledge, following industry trends, and growing your expertise.
In comparison to some other programming languages, C++ is frequently thought of as being faster for a number of reasons resulting from its design decisions and features. The following are some important elements that support C++'s reputation for being quick:
1. Performance-Oriented Design: C++'s original design prioritized performance. It is a statically typed, compiled language that enables precise management of hardware resources and direct memory manipulation. Machine code, which may be directly executed by the computer's hardware, is normally how C++ programs are generated, resulting in effective and quick execution.
2. Memory Control: C++ offers explicit memory allocation and deallocation, pointers, and other features that enable manual memory control. As a result, unnecessary overhead is reduced and programmers may precisely control how and when memory is created and deallocated. Faster execution and less memory usage can result from effective memory management.
3. Support for inline functions and function templates is provided by C++. To avoid the overhead of function calls, inline functions are expanded at the location of the call. Generic programming is made possible via templates, which also allow the compiler to provide customized code for various types without the requirement for runtime polymorphism. Code that is optimized and executed more rapidly can be produced via the use of inline functions and templates.
4. small Runtime Environment: Compared to languages that rely on virtual machines or interpreters, C++ offers a small runtime environment. As a result, runtime environment overhead such as memory allocation for trash collection or bytecode interpretation is reduced. Because C++ programs don't require a complex runtime environment, their performance is enhanced because they can operate more closely with the hardware.
5. Low-Level Language capabilities: C++ comes with low-level language capabilities that let users interface directly with system and hardware resources. Programmers can develop code that is highly optimized for particular hardware architectures because to features like raw pointers, bitwise operations, and access to low-level system APIs. The execution of code may be accelerated and made more effective with this degree of control over low-level details.
6. Compilers that can be optimized: C++ compilers are renowned for their sophisticated optimization methods. In order to produce highly efficient machine code, modern compilers carry out a variety of optimizations, including inlining functions, loop unrolling, constant propagation, and dead code eradication. These improvements can make C++ applications perform substantially better than code produced by less optimized compilers or interpreted languages, making them faster.
Although C++ has the potential for excellent performance, it's crucial to remember that a program's actual speed depends on a variety of factors. In order to get optimal performance in C++, a number of factors must come together, including algorithmic effectiveness, data structures, code design, and the programmer's skill. Low-level programming ideas must be thoroughly understood in order to write good code and make effective use of the language's performance-oriented features.
The primary themes in C++ cover a wide range of ideas and elements that are necessary for comprehending and efficiently using the language when programming. The following are some of the major C++ topics:
1. Syntax and Basic principles: Writing effective and correct programs requires a thorough understanding of C++'s syntax and fundamental principles. Variable declaration and initialization, operators, control structures (if-else, loops), functions, and input/output operations are some of the subjects covered in this.
2. Data Types and Variables: The C++ programming language comes with a number of built-in data types, including integers, floating-point numbers, characters, and Booleans. It is crucial for handling data in C++ applications to comprehend data types, their sizes, and ranges as well as the procedures for declaring and using variables.
3. Pointers and Memory Management: Pointers make it possible to directly modify and access memory addresses. Pointer arithmetic, dynamic memory allocation with 'new' and 'delete', memory deallocation, and memory management strategies like RAII (Resource Acquisition Is Initialization) are all related topics.
4. Object-Oriented Programming (OOP): OOP is a core idea in the object-oriented programming language C++. Classes, objects, encapsulation, inheritance, polymorphism, constructors, destructors, access specifiers, and object relationships are some of the topics covered by OOP.
5. Templates and Generic Programming: C++ templates allow for generic programming, allowing for the creation of code that can operate on a variety of data types. Function templates, class templates, template specialization, template metaprogramming, and template libraries like the Standard Template Library (STL) are all topics connected to templates.
6. Standard Library: The C++ Standard Library offers a collection of libraries and containers that provide functionality for typical programming tasks. Input/output operations, string manipulation, containers (like vectors, lists, and maps), algorithms (like sorting and searching), and utilities (like smart pointers and exceptions) are all tied to the Standard Library.
7. Exception Handling: In C++ applications, exception handling enables the management and recovery from runtime failures. Try-catch blocks, throw clauses, exception classes, exception propagation, and resource cleanup using RAII are among the topics covered.
8. Handling Files: C++ has built-in tools for reading from and writing to files. File streams, opening and shutting files, reading and writing data, and handling file-related errors are all topics in file handling.
9. Memory Management and Smart Pointers: C++ applications must effectively manage their memory. Memory management topics include the use of smart pointers to handle memory deallocation automatically, memory allocation algorithms, managing dynamic memory, and stack and heap memory.
10. Advanced topics: Advanced subjects in C++ include lambda expressions, move semantics, operator overloading, function and class templates, type traits, and metaprogramming approaches. They also cover multithreading and concurrency.
These subjects offer a thorough basis for comprehending and using C++ programming. It is possible for programmers to create robust, effective, and maintainable C++ code by mastering these concepts and topics.
C++ has a number of essential components that add to its strength, adaptability, and appeal as a programming language. Let's explore these characteristics in greater detail:
1. Support for object-oriented programming (OOP): C++ enables programmers to organize their code using objects and classes. In order to organize, modularize, and reuse code, objects combine data (attributes) and behaviors (methods) into a single unit. Classes, inheritance, and polymorphism are features that make it easier to apply OOP concepts in C++, allowing programmers to create intricate systems and accurately model real-world objects.
2. Generic programming is possible in C++ thanks to the use of templates. Generic classes and functions that can operate on several data types can be created using templates. Templates offer a method for flexible code reuse by parameterizing types and algorithms. This strategy enables programmers to create generic algorithms that can run without duplicating code on a range of data types. A strong component that adds to C++'s adaptability and flexibility is generic programming.
3. Strong Type Checking: C++ requires strong type checking, which improves program dependability and makes it easier to find mistakes at the time of compilation. The compiler makes sure that data types are used appropriately and consistently for each expression and variable in C++. By ensuring that incompatible operations or assignments are detected as errors before the program is performed, strong type checking helps prevent type-related issues and encourages safer programming practices.
4. Operator Overloading: The C++ language provides operator overloading, which enables the definition of operators like +, -, *, and / for user-defined types. With the help of specially defined operations, this feature allows objects to behave naturally. For instance, items of a class can be put together using a syntax resembling built-in types by overloading the + operator. Operator overloading makes working with user-defined types simple and expressive, improving the readability and usability of the code.
5. Handling Exceptions: C++ has a strong exception handling system that enables programmers to handle and react to exceptional circumstances in their code. During program execution, exceptions are used to manage errors or other unforeseen circumstances. In order to capture and gracefully manage exceptions, particular code blocks (try-catch blocks) might be specified. This makes it easier to separate error-handling code from the program's main flow and guarantees proper resource cleanup in the event of exceptions.
6. Templates: A key component of C++ that enables generic programming are templates. Without duplicating code, developers can use templates to create functions or classes that deal with various data types. Generic functions can be created using function templates, whereas generic classes can be defined with greater freedom using class templates. By enabling the creation of type-independent algorithms and data structures, templates improve the efficiency and flexibility of C++ programs as well as the reusability of code.
In conclusion, support for object-oriented programming (OOP) and generic programming, rigorous type checking, operator overloading, exception handling, and the effective usage of templates are some of the major characteristics of C++. Together, these capabilities make C++ a flexible language that can handle challenging software development jobs in a variety of domains. Programmers can build C++ code that is effective, modular, and flexible by successfully understanding and leveraging these capabilities."
A derived class may offer its own implementation of a function that is already specified in its base class in C++ by using the concept of overriding. When a base class function is designated as virtual, a derived class function with the same signature may replace it. As a result, the derived class can adapt the behavior of the base class function to suit its own needs.
Think about a situation where a base class defines a virtual function to grasp overriding. The virtual keyword denotes that descendant classes may override the function. A derived class can redefine the virtual function with the same name, return type, and parameter list when it inherits from the base class. An overridden function is one that has been redefined in the derived class.
The following steps are involved in the overriding process:1. Base Class Declares a Virtual Function: The base class declares a virtual function that will be overridden by derived classes. The behavior of the function is denoted by the virtual keyword.
2. The Derived Class Redefines the Virtual Function: The Derived Class redefines the virtual function with the same name, return type, and parameter list. Although it is not required, the function in the derived class should likewise be defined as virtual.
3. Invocation and Polymorphic Behavior: The implementation of the derived class is called at runtime when an object of the derived class is created and the overridden function is called using a pointer or reference to the base class. The proper function implementation is chosen based on the actual type of the object being referenced in this situation, which is known as polymorphic behavior.
It's crucial to remember that for overriding to take place, the function signatures (name, return type, and parameters) between the base and derived class functions must exactly match. The derived class function won't replace the base class function if the signatures don't match because it will be regarded as a separate function.
Object-oriented programming can be flexible and extensible thanks to overriding. It gives the derived classes the ability to customize or alter the virtual function's behavior in the base class. This is especially helpful when distinct derived classes want various implementations of a base class-defined common action.
A derived class's ability to offer its own implementation of a virtual function declared in its base class is known as overriding in C++. The proper function implementation is chosen based on the actual type of the object being referred, enabling polymorphic behavior. In object-oriented programming, overriding makes code expansion and modification easier.
In C++, a namespace is a technique for classifying similar names (identifiers) into different scopes. It offers a mechanism to organize code in a modular and organised style and helps prevent naming conflicts.
A namespace in the C++ programming language is a named declarative area that can house named variables, functions, classes, and other entities. Namespaces offer a mechanism to bring together similar code components under a single name. This aids in separating items with the same name that may exist in many namespaces or with distinct global scopes.
The 'namespace' keyword is used to define a namespace, and then the desired namespace name is followed by a block of code contained in curly braces. For instance:
namespace MyNamespace { // Declarations and definitions }
Variables, functions, classes, and even other nested namespaces can all be created and specified inside a namespace. Code that is a part of the same namespace or explicitly specifies the namespace can use these entities because they are available within the namespace itself.
Namespaces provide the following advantages:
1. Preventing Naming Conflicts: Naming conflicts can be avoided by grouping related code elements into namespaces. Entities with the same name can exist in many namespaces as long as they are declared in distinct namespaces. When merging various libraries or code modules, this maintains code clarity and prevents conflicts.
2. Coding organization and structure: Namespaces offer a method for classifying code into logical categories. It enables programmers to organize code according to functionality, goal, or module. As related items are grouped together and are simple to find and comprehend, this arrangement improves the maintainability and readability of the code.
3. Encapsulation and Modularity: Namespaces offer a type of encapsulation by enabling entities inside a namespace to be hidden from other namespaces or the global scope. This encourages information concealment and lowers the possibility of entities being accessed or altered without authorization. By making it easier to create self-contained code units that can be quickly reused or shared, namespaces also enhance modularity.
4. Qualified and Unqualified Access: Names that are qualified or unqualified can be used to access entities inside a namespace. Unqualified access, often known as accessing entities after a 'using' directive or directly using their names within the same namespace, is referred to as such. To access entities from another namespace, you must first declare the namespace and then the scope resolution operator '::'.
The 'namespace' alias syntax in C++ allows for the aliasing of namespaces as well. When dealing with big or nested namespaces, this enables the creation of names for namespaces that are shorter or more understandable.
In C++, namespaces offer a means of classifying, structuring, and encapsulating code. They enable qualified or unqualified access to entities within a namespace, assist in avoiding naming conflicts, and enhance the modularity and maintainability of the system. Namespaces allow programmers to create well-structured, modular code that is simple to reuse and combine with other libraries or code modules.
A member function of a class that is declared in the base class of C++ is referred to as a virtual function and may be overridden by derived classes. When a function is designated as virtual, it means that it is meant to be overridden in derived classes and that the proper function implementation should be decided dynamically at runtime based on the actual type of the object being referred.
The virtual function mechanism supports dynamic binding, also known as late binding, which refers to the process of resolving a function call at runtime based on the actual type of the object being referenced, as opposed to the type of the pointer or reference that was used to access it. As a result, many derived classes can offer their own implementations of the same virtual function, enabling polymorphic behavior.
The virtual keyword must be appended to the function declaration in the base class in order to declare a virtual function. For instance:
class Base { public: virtual void someFunction() { // Base class implementation } };
A function in a derived class is designated as virtual when it inherits from the base class and redefines the virtual function with the same signature. To clearly state that a function is meant to replace a virtual function from the base class, use the override keyword (C++11 and later).
class Derived : public Base { public: void someFunction() override { // Derived class implementation } };
The actual function implementation that will be performed when a virtual function is called via a base class pointer or reference is chosen at runtime based on the dynamic type of the object. This enables various behavior based on the type of the derived class in question.
Base* ptr = new Derived(); ptr->someFunction(); // Calls the overridden function in the Derived class }
The function call is resolved depending on the type of the pointer or reference at compile-time if a function is not designated as virtual. This process is referred to as static binding or early binding. In this instance, regardless of the actual object type, the function implementation is chosen based on the type of the pointer or reference.
A key feature of object-oriented programming (OOP) in C++ is the usage of virtual functions, which promote polymorphism, flexibility, and code expansion. It allows derived classes to provide specialized implementations based on their unique requirements while allowing the base class to define a common interface. In order to establish hierarchical relationships between classes and provide dynamic behavior based on the actual object type, virtual functions are crucial.
It's crucial to remember that because dynamic dispatch is required, virtual functions have a tiny performance overhead compared to non-virtual functions. However, in most cases, this penalty is minimal, and the advantages of polymorphism and code flexibility outweigh the performance cost.
A member function that is declared in a base class as a virtual function in C++ can be overridden by derived classes, to put it briefly. It permits dynamic binding and enables runtime determination of the proper function implementation based on the actual object type. In C++, virtual functions are essential to object-oriented programming and provide support for polymorphic behavior.